In the first post we discussed the basics of images and color models. Now although most of the processing and learning is done on images, we often have to extract the individual images from a video. For example, face recognition or object tracking tasks are generally done on video streams. In this post, we'll see how we can work with video files/streams in Python. We'll also look into using our phones as cameras just in case you don't have a webcam or if it's a potato(like mine).
Working With Videos In OpenCV
Reading Videos
OpenCV offers the cv2.VideoCapture
class that can be used to work with videos. The VideoCapture(source)
constructor takes the video source as the argument. This argument can take many forms:
0
or1
: Theseint
values correspond to the primary and secondary camera on your system. If there's only one camera1
will return a blank window."path"
: We can also path to a local video file."IP_address"
: Another possible video source can be a live stream from a remote CCTV camera or IoT device.
Whatever video source we work with, the video is read frame by frame by the VideoCapture.read()
method. read()
returns a tuple of two values, first is a boolean value that signifies if the frame has been read successfully, and the second is the frame.
Let's try it out!
import numpy as np
import cv2
# create the VideoCapture object with the relevant source argument
cap = cv2.VideoCapture(0)
# read through the frames sequentially
while True:
ret, frame = cap.read()
if ret:
smaller_frame = cv2.resize(frame, (0, 0), fx=0.5, fy=0.5)
cv2.imshow('IP Cam Stream', smaller_frame)
# exit if the video finishes
else:
break
# checking if the ESC key is pressed to break out of loop
if cv2.waitKey(1) == 27:
break
#releasing the camera resource so other processes can use it
cap.release()
cv2.destroyAllWindows()
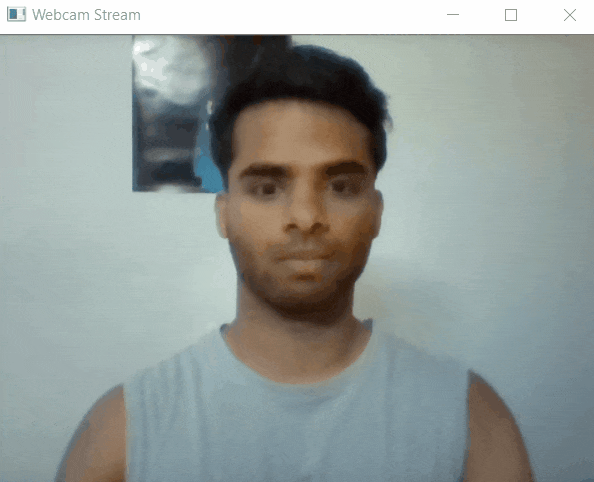
Now, with a local file.
cap = cv2.VideoCapture("video\clownfish.mp4")
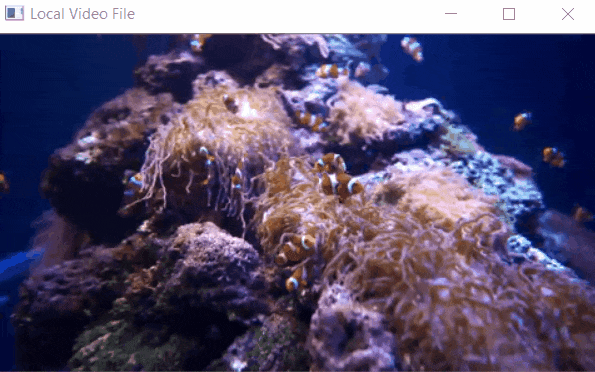
Using Your Phone as the Video Source in OpenCV
What if you don't have a webcam? Or just a low-res one? No worries, you can just use your phone! There is a wide range of apps—for both Android and iOS—that allow you to access your phone's camera output over the internet. You just need to install the app, open it, give it the camera permissions and you're good to go. It's actually scary to see how easy it is!
I have personally used DroidCam and IP Webcam and I have mentioned the IP address routes for both of them in the comments.
# DroidCam: "IP address:PORT/mjpegfeed"
# IP Webcam: "IP address:PORT/video"
cap = cv2.VideoCapture("https://192.168.1.4:8080/video")
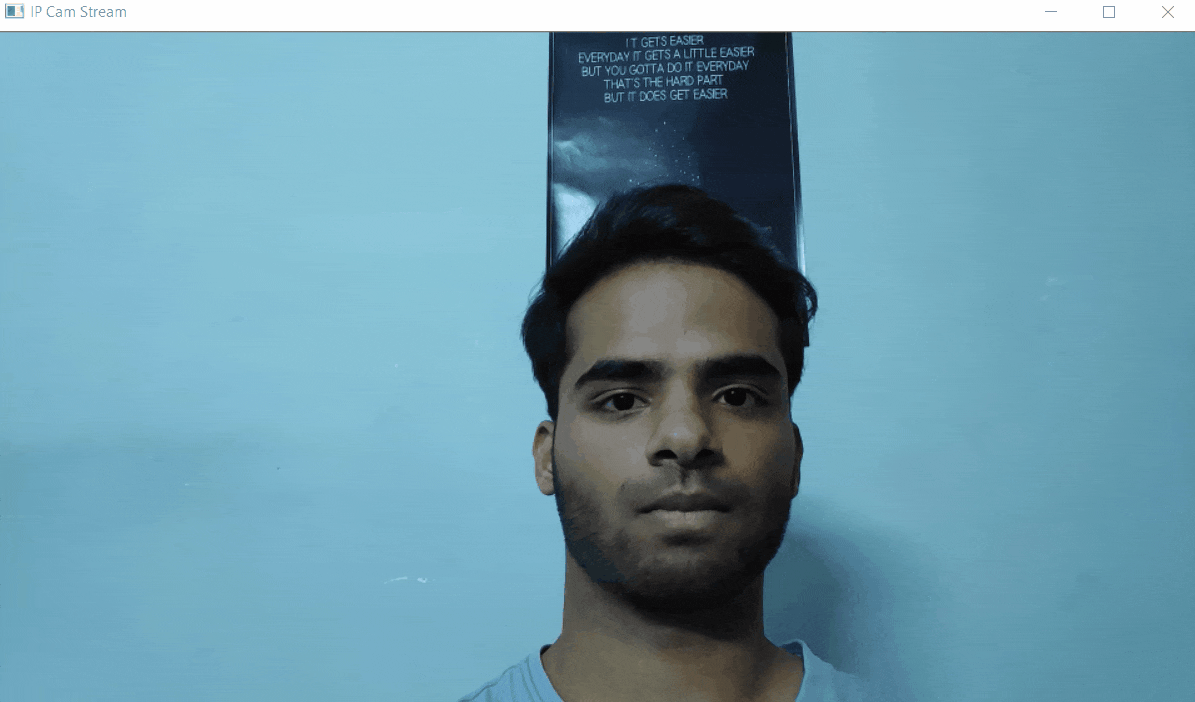